C++ FOUNDATION WITH
DS + ALGO
What you’ll learn ?
- Learn Second most used and powerful language in the Industry C++.
- Learn C++ using a proven curriculum that covers more material than most C++ university courses
- Learn various Popular Data Structures.
- Learn Algoithms.
- Entry Level Knowledge to become a Software Engineer.
This course is designed to take you from zero to hero, For absolute beginner in programming. This certified training is designed by Industry experts to make students Internship and Job ready.
*Since this program is for absolute beginner hence no pre-requisite of programming is required.
*Junior Programmer
*Software Developer
*Game Programmer
*Programming Architect
*C++ Analyst
*Unix Shell Scripting
*Backend Developer
*Embedded Engineer
PROGRAM INDEX
Introduction – History of C++, Assembler, Compiler, Linker & Loder, Execution Process.
various softwares available, Code-bloks Installation, Online Compilors.
Basic requirements to write a program – Comments, #include Statement, Printf & Scanf, Displaying Output, reading Input from terminal.
Writing first Program, Datatypes, Data types, Primitive Data types, Variables,
Introduction to Arrays, Arrays Declarations, challenge – Adding all Elements of Array, Finding Max element from Array,
Introduction to Pointers,
Introduction to Strings, Reading and Writing String, Length , Concatenate and Copy,Substring and Compare,Tokeniser and To Integer.
Introduction to functions, Function for Adding 2 numbers,Function for Finding maximum of 3 numbers,
introduction to OOPS, Principles of Object-Oriented Programming, Class vs Objects, Writing a Class in C++, Pointer to an Object, Philosophy Behind Data Hiding,Data Hinding in C++ (Accessors and Mutators), Accessors and Mutators.
Inheritance with example, Constructors in Inheritance, isA and hasA, Access Specifiers, Types of Inheritance, Ways of Inheritance, Generalization and Specialization.
Exception Handling, Exception Handling Construct, Throw and Catch Between Functions, All About Throw & Catch.
Converting Binary to Octal to hexadecimal.
Big O nortation, Reading declaration, controller OS, Pass by value and refference revise, requirements to write a program, Stacks, Queues, Linked List, Trees
LIFO, FIFO, user applications, array vs stacks, push & pop, applications, reverse.
LIFO, FIFO, user aplications, array vs queus, Insert, Delete, application, reverse.
Infix, Postfix, Prefix, Local variables, psudo code, palindrome, paranthesis, conversion, search operation (null, max, min) [psudo code & c code], reverse, application.
Singly, Doubly, Circular.
array vs Linked list,
node creation, Insert front & rear, Delete
Display, pvg for Insertion, Deletion, Front and rear end.
Doubly Linked List – Insertion front & rear end, delete, display
Implementation to stack, queue
Print middle node, kth node, search multiples, max.
concatenation of linked list,
reverse linked list.
Leaf node, Root node
different trees – ordinary, binary, strictly binary, complete binary, binary search tree, building binary tree.
Tree Traversals – Pre-order, In-order, Post order.
Insert node.
Recursion, Sorting, Searching, BFS+DFS,Dynamic Programming.
- Introduction to Algorithms, Recursion Introduction,
- Stack Overflow,
- Anatomy Of Recursion, Exercise: Factorial
- ,
Fibonacci, Recursive VS Iterative, Recursive VS Iterative, When To Use Recursion, Exercise: Reverse String With Recursion.
- Sorting Introduction
- The Issue With sort()
- Sorting Algorithms
- Bubble Sort
- Selection Sort
- Dancing Algorithms
- Insertion Sort
- Merge Sort and O(n log n)
- Stable VS Unstable Algorithms
- Quick Sort
- Which Sort Is Best?
- Heap Sort
- Radix Sort + Counting Sort
- Searching + Traversal Introduction
- Linear Search
- Binary Search
Graph + Tree Traversals
- BFS Introduction
DFS Introduction
BFS vs DFS
Resources: BFS vs DFS
- Exercise: BFS vs DFS
- Solution: BFS vs DFS
- breadthFirstSearch()
- breadthFirstSearchRecursive()
- PreOrder, InOrder, PostOrder
- depthFirstSearch()
- Optional Exercise: Validate A BST
- Graph Traversals
- BFS in Graphs
- DFS in Graphs
- Dijkstra + Bellman-Ford Algorithms
- Searching + Traversal Review
Hash Tables Introduction
Hash Function, Hash Collision, Hash Tables In Different Languages
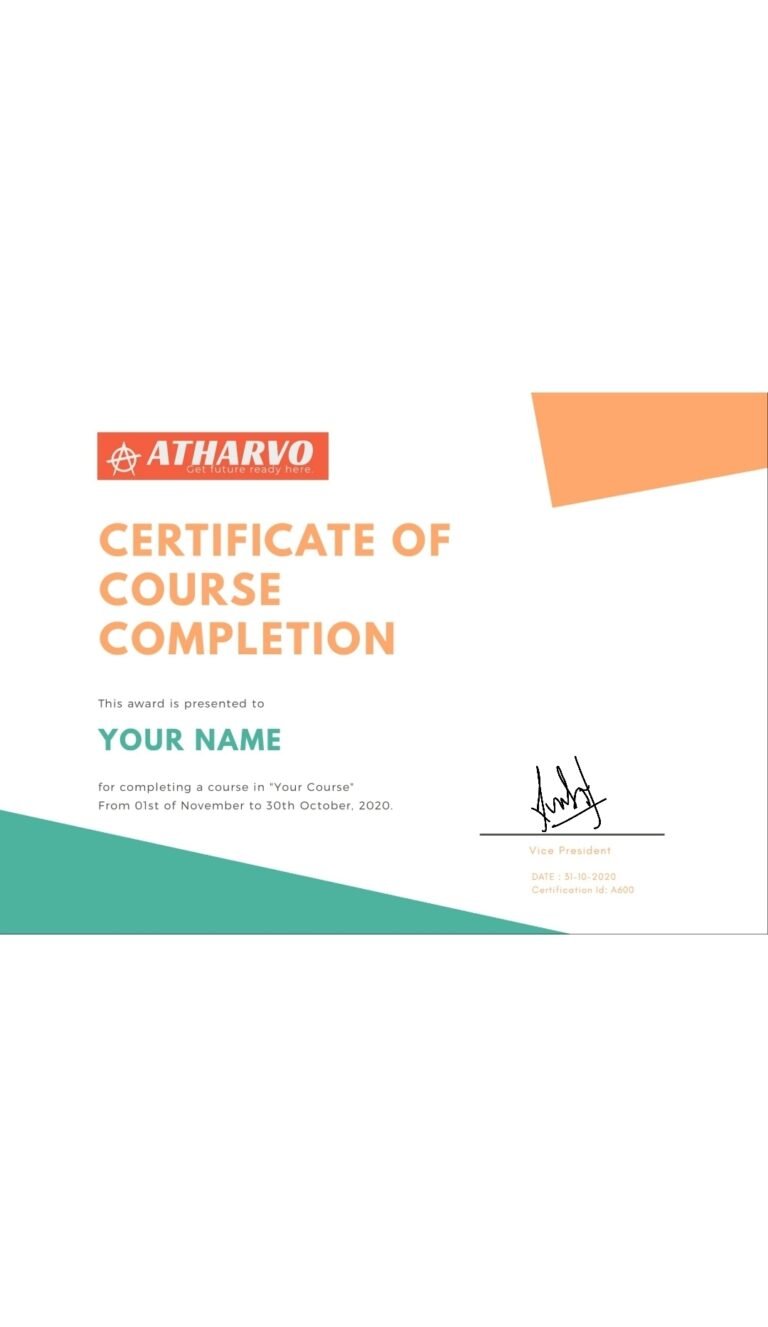

C E T I F I C A T E S
With what you have gained including experience, get the shot in the arm for your career prospects and interviews with skills and certificates to back your talk and stand tall among others in the crowd.
PRICING plan
Our courses are offered at the best rates in the industry that fit perfectly in your budget and offer you the best services.
Self Placed
₹2500
Pre-enrolment price ₹1000
- 25+ hours of classes
- Curated reference and study material
- Course Completion Certificate
- Performace Certificate
- Exclusive life time access to forum community
- 24/7 Mentor Support
- Atharvo Membership
Mentor Support
₹5000
Pre-enrolment price ₹1000
- 40+ hours of classes
- Curated reference and study material
- Course Completion Certificate
- Performace Certificate
- Exclusive life time access to forum community
- 24/7 Mentor Support
- Atharvo membership
Advanced
₹13000
Pre-enrolment price ₹1000
- 50+ hours of classes
- Curated reference and study material
- Course Completion Certificate
- Performace Certificate
- Exclusive life time access to forum community
- 24/7 Mentor Support
- Atharvo Membership
Frequently Asked Question
C++ is the one of the top 5 coding languages and 2nd most used language in IT Industry, and also it is the base Backend and gaming Industries.
So, for a begginer who wants to enter IT Industry it is the best choice.
One should know Datastructures and Algorithms to get Internship/Job in any IT company, It is the primary expectation from any company from a Student/Fresher.
The answer is yes, for any student/fresher who wants to start there career in IT industry persuing this begginer program is more than enough to get it into an IT company.
For this program the total fees is Rs.5000 but students know need to pay entire fee at once so, they can book there slot by paying Pre-enrolment amount Rs.1000 to book there slot which included in the main fees, Prior to the commencement of the program our executive will contact you to pay remaing amount Rs.4000 at that time you need to pay the remaining amount.
Pre-recorded: The pre-recorded sessions are hosted on different platforms you will get access to it once the program commence.
Live Sessions: The live sessions are hosted via one of the video conferencing platforms such as ZOOM.US or Webex or Google Meet.